class: center, middle # Abstract Syntax Trees and Tree Traversal _CMPU 331 - Compilers_ --- # Recap: Stages of Compilation 1. Lexical Analysis 2. Parsing 3. Semantic Analysis 4. Optimization 5. Code Generation 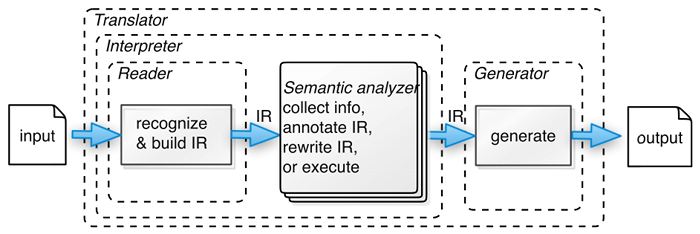 (source: _Language Implementation Patterns_ by Terence Parr) --- # Stages of Compilation * Read plain-text code, and roll it into a tree * Clean up the tree * Flatten the tree back into plain-text code 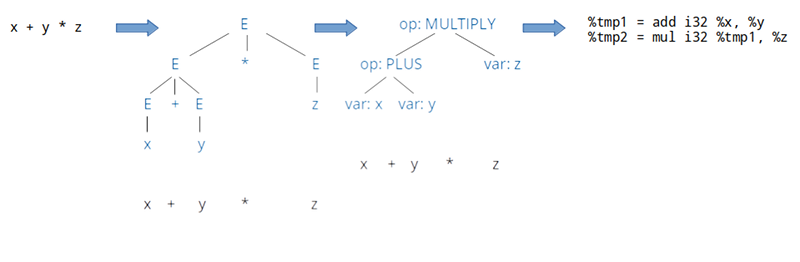 --- # Linked Lists Remember linked lists? * Each node in the list contains data, and a pointer to the next node  (source: _Linked Lists_ on Wikipedia) * Iterating over the linked list means visiting each node in order * For example, to sum the value of all nodes: ``` while node != null: total = total + node.value go on to next node ``` --- # Tree Traversal * Trees are similar to linked lists * Each node contains data, and can link to multiple other nodes * Iterating over a tree means visiting each node in order 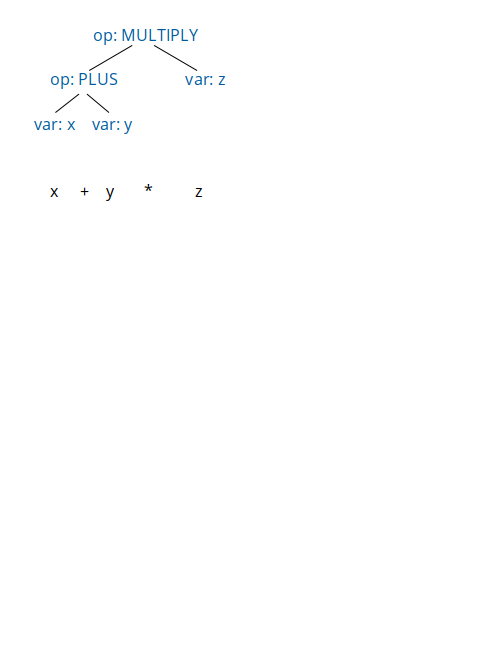 --- # Tree Traversal in Compilers * Parsing is about building trees * Semantic analysis and code generation is about traversing trees * Look at every node in the AST and do _something_ * Semantic analysis: check the node data, and throw an error if it's not right * Code generation: write plain-text code for the node 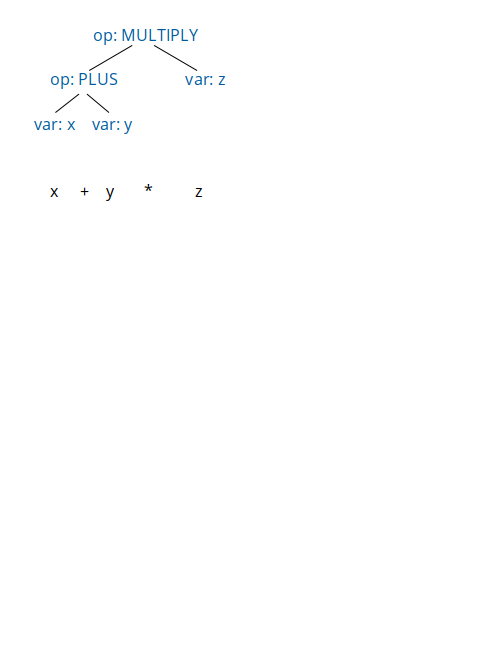 --- # Design Patterns * A general, reusable solution to a commonly occurring problem in software design * Can be implemented in any language * Book: [Design Patterns: Elements of Reusable Object-Oriented Software](https://en.wikipedia.org/wiki/Design_Patterns) (1994) by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, sometimes called the "Gang of Four" * _Decorator Pattern_: dynamically adds/overrides behaviour in an existing method of an object * _Factory Method Pattern_: creates objects, but automatically chooses which class to create * _Visitor Pattern_: separates an algorithm from an object structure by moving the hierarchy of methods into one object --- # Visitor Pattern * One of the design patterns from the original Gang of Four book * It's just a way of visiting all the nodes in a tree * The tree has a bunch of nodes of different types * The "visitor" is a class that knows how to visit all the nodes in the tree * For each type of node, the visitor implements the "do something" part * It's one of the simplest ways of implementing tree traversal in a compiler ```python class ASTVisitor: def visit_BinOp(self, node): # do something with BinOp nodes def visit_Integer(self, node): # do something with Integer nodes ``` * But, not the only way, there are plenty of alternatives